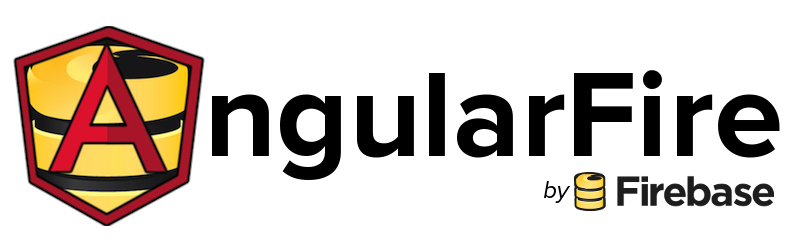
One of the cool features in AngularJS is the two-way data binding. When you change a property of an object, the value is instantly changed in every view or service that is using this property. A while ago, I saw a video about the combination of AngularJS and Firebase. This combination makes it possible to create three-way data binding in AngularJS.
What is Firebase?
Firebase is an online service that provides an API to store and sync data in realtime. This means that when the data is saved, all connected clients will retrieve the latest data without reloading the page. You can see Firebase as a database service with some extra features like authentication. The cool thing about this service is that you don’t need to create a database schema. You can create collections just by specifying a path to the database in the URL. An additional package ‘AngularFire’ is available for integration with AngularJS.
The world’s easiest guestbook
Getting started
A good way to start a test project is by using Yeoman. Both AngularJs and AngularFire have a generator that will create your basic application in no time. Just run following commands and follow the wizard:
$ yo angular
$ yo angularfire
$ grunt serve
The view
<div class="jumbotron">
<h1>'Allo, 'Allo!</h1>
<p class="lead">
Please leave a message if your like my page!
</p>
<div class="form-group">
<input ng-model="input.name" placeholder="Name" class="form-control" />
</div>
<div class="form-group">
<textarea ng-model="input.message" class="form-control" placeholder="Message"></textarea>
</div>
<p><a class="btn btn-lg btn-success" data-ng-click="addMessage()">Splendid!</a></p>
</div>
<div class="messages" ng-cloack="">
<div class="message" id="message-{{ message.$id }}" ng-repeat="message in messages | orderByPriority | reverse">
<div class="name">{{ message.name }}</div>
<div class="content">
{{ message.message }}
</div>
</div>
</div>
Initializing your firebase collection
The AngularFire package provides some ways to interact with Firebase. The easiest way to connecto to a collection is by using the syncData service. We can create the connection with one line of code:
angular.module('app')
.controller('MainCtrl', function ($scope, syncData) {
$scope.messages = syncData('messages');
});
Add a message
Now that we set up the connection, we want to add messages to the collection. This part is very easy: you just pass a JSON object to the collection:
$scope.input = {
message: '',
name: ''
};
$scope.addMessage = function()
{
$scope.messages.$add({
name: $scope.input.name,
message: $scope.input.message,
timestamp: Date.now()
});
$scope.input.message = '';
}
Sort messages in reverse order
When you want to display the messages in reverse order you should create one extra filer called reverse
. This filter is allready written in one of the example AngularFire projects. You can find the code over here.
A quick way to create a custom filter is by using the Yeoman angular:filter generator:
$ yo angular:filter reverse
Hosting
It is also possible to use Firebase hosting. A custom CLI tool is provided an can be installed through NPM:
$ npm install -g firebase-tools
The next step is to create a firebase configuration file. This can be done with the command:
$ firebase init
Note: The destination folder should be dist
The next step is to deploy the application. This is also very easy:
$ grunt build
$ firebase deploy
The sample application is now available at: Firebase App.